Understanding programming languages is key to modern software development. Whether you’re choosing a high-level or low-level language, the right tools like IDEs, compilers, and interpreters can streamline the process. This guide dives into how these technologies impact your coding workflow.
What Do Programming Languages Do?
Programming languages serve as the bridge between humans and computers, allowing us to communicate instructions and create software applications. There are two primary categories of programming languages: high-level and low-level. Understanding the differences between these languages, along with the tools used to translate them into machine code, is essential for software development.
Mastering Programming Languages: From High-Level to Low-Level
High-level languages are designed to be human-readable and easier to write and understand, allowing developers to focus on writing programs rather than dealing with complex machine instructions.
These languages including Python, Java, and C# provide significant abstraction, hiding details of hardware and memory management. Developers often use integrated development environments (IDEs) to write and optimize these higher-level languages.
High-level languages
High-level programming languages simplify the coding process by abstracting away the complexities of machine languages. This makes it easier for developers to focus on solving problems and building software rather than managing low-level details like memory management and binary code.
Advantages of high-level languages:
- Readability: Easier to read, write, and maintain due to more natural syntax.
- Portability: Programs written in these languages can run on multiple platforms with minimal changes.
- Abstraction: Hide the intricacies of hardware and operating systems, allowing developers to focus on logic.
Disadvantages of high-level languages:
- Performance: May be slower than low-level languages due to additional layers of abstraction.
- Limited Control: Less direct control over hardware resources, which may limit performance optimization in certain cases.
Low-level languages
Low-level languages like assembly language and machine languages offer developers direct control over hardware, making them suitable for performance-critical applications. These languages are less abstract and closer to the machine’s native binary code.
Advantages of low-level languages:
- Performance: Programs written in low-level languages can achieve higher performance due to direct hardware manipulation.
- Control: Provides better control over system resources, such as CPU and memory.
Disadvantages of low-level languages:
- Complexity: Writing code in low-level languages is more challenging and time-consuming due to its complexity.
- Portability: Code written in these languages is less portable, often tied to specific hardware architectures.
Why Translators Matter: Compilers, Interpreters, and Assemblers Explained
Translators play a crucial role in converting human-readable code into machine languages that computers can execute. These translators, whether compilers, interpreters, or assemblers, ensure that programs run smoothly across different platforms and hardware.
Assembly Language and Translators
Assembly language is a low-level language that translates human-readable instructions into machine code through an assembler. It offers more readability than pure machine code but still requires an understanding of hardware and memory management.
An assembler is a translator that converts assembly language code into machine code. It replaces mnemonic instructions with their corresponding machine code equivalents.
Compilers and Interpreters
A compiler translates the entire source language into machine code before execution. This can lead to optimized performance but makes the development cycle longer. An interpreter, on the other hand, translates and executes the code line-by-line, offering immediate feedback and easier debugging but at the cost of slower execution.
Advantages and Disadvantages of Compilers and Interpreters
Compilers:
- Faster Execution: Once compiled, the program runs faster as it doesn’t need further translation.
- Optimization: Compilers can optimize the code for better performance.
- Distribution: Compiled programs can be distributed as standalone executables.
- Slower development cycle: Compiling large programs can take time, slowing down the development process.
Interpreters:
- Faster development cycle: Interpreters allow for immediate feedback, making it easier to test and debug code.
- Platform independence: Interpreted programs can often be run on different platforms without recompilation.
- Slower execution: Interpreted programs generally execute slower than compiled programs.
- Dependency on interpreter: The interpreter must be installed on the target system for the program to run.
How IDEs Supercharge Software Development: Tools You Need
An integrated development environment (IDE) is an essential tool for modern software developers, offering an all-in-one solution for writing, debugging, and testing code. IDEs enhance productivity by providing features like syntax highlighting, code completion, and error diagnostics, all within a single interface.
Common IDE features include:
- Code editors: Provide syntax highlighting, code completion, and other features to assist in writing code.
- Run-time environment: Allow you to execute your code and debug it.
- Translators: Integrate compilers or interpreters for building and running your programs.
- Error diagnostics: Highlight errors and provide suggestions for fixing them.
- Auto-completion: Suggest code snippets and complete statements automatically.
- Auto-correction: Automatically fix common coding errors.
- Prettyprint: Format code for better readability.
We did a good comparison of tools here. Check it out!
Other Essential Development Tools
In addition to IDEs, there are other key development tools that every software developer should know about. These tools complement your programming environment and improve efficiency, collaboration, and code quality.
Version Control Systems (VCS)
Version control systems like Git are critical for tracking changes in your codebase. They allow multiple developers to collaborate on a project without overwriting each other’s work, providing a complete history of changes.
Benefits:
- Collaboration: Enables seamless collaboration among team members.
- History Tracking: Maintains a detailed history of changes, making it easier to revert to previous versions.
- Branching and Merging: Supports feature development without affecting the main codebase until changes are approved.
Build Automation Tools
Build automation tools like Maven, Gradle, and Make streamline the process of compiling code, running tests, and packaging applications. These tools automate repetitive tasks, reducing the risk of human error and improving efficiency.
Benefits:
- Consistency: Ensures that software builds are consistent and repeatable.
- Efficiency: Automates time-consuming tasks like compilation and testing.
- Integration: Often integrates with continuous integration (CI) systems for automated deployment.
Debugging Tools
Effective debugging tools are essential for identifying and fixing issues in your code. While many IDEs come with built-in debuggers, stand-alone debugging tools like GDB (GNU Debugger) can provide additional control and depth for specific languages or environments.
Benefits:
- Error Identification: Helps pinpoint issues in your code.
- Real-Time Monitoring: Tracks variable states and system performance during execution.
- Step-Through Execution: Allows developers to execute code line-by-line to find the source of errors.
Choosing the Right Programming Language and Tools for Your Project
When choosing a programming language, it’s important to consider the requirements of your project, including performance, portability, and ease of development. Higher-level languages like Python or Java are ideal for general software development because they balance readability and performance. For system-level programming or tasks requiring fine control over hardware, low-level languages such as C or assembly language may be more appropriate.
Factors to consider:
- Project Complexity: For larger, more complex projects, high-level languages and IDEs streamline development and debugging.
- Performance Needs: Low-level languages provide greater control for performance-critical applications.
- Cross-Platform Requirements: If your project needs to run on multiple platforms, choose a high-level language with strong portability.
Optimizing Coding Workflow with Translators and IDEs
Streamlining your coding workflow means making the most of tools like IDEs and translators (compilers and interpreters). By automating repetitive tasks, providing real-time error checks, and offering suggestions through features like auto-completion, an IDE can improve both the quality and efficiency of your code.
- Use a command line interface in combination with IDEs for faster project setup and deployment.
- Leverage version control systems like Git to track changes and collaborate effectively.
- Integrate build automation tools to streamline the compilation and testing of large codebases.
Understanding the different types of programming languages, translators, and IDEs is essential for effective software development. By choosing the right tools and techniques, developers can improve their productivity and create high-quality software applications.
Quick FAQ for “Programming Languages Guide”
- What is the difference between high-level and low-level programming languages?
High-level languages are designed for easier readability and focus on problem-solving, while low-level languages give you direct control over hardware but are more complex. - Why are translators important in programming?
Translators convert human-readable code into machine code, ensuring that the instructions can be executed by computers efficiently. Without them, software wouldn’t function. - What are the benefits of using an IDE for software development?
IDEs offer built-in tools like code editors, debuggers, and compilers, streamlining the software development process and improving productivity by automating repetitive tasks. - How do compilers and interpreters differ in programming?
Compilers convert entire code into machine code at once, improving performance, while interpreters execute code line-by-line, allowing for quicker testing but slower execution.
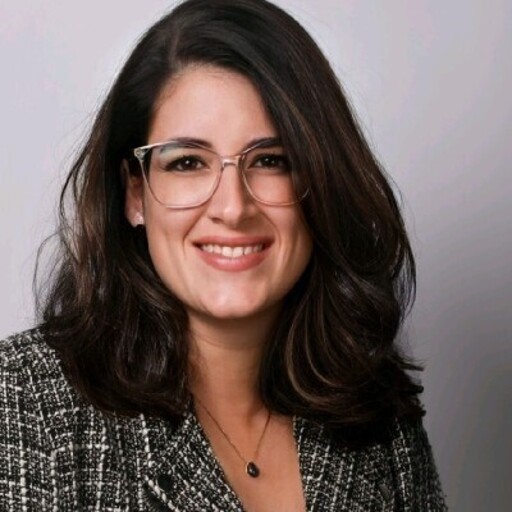
International Marketing Leader, specialized in tech. Proud to have built marketing and business generation structures for some of the fastest-growing SaaS companies on both sides of the Atlantic (UK, DACH, Iberia, LatAm, and NorthAm). Big fan of motherhood, world music, marketing, and backpacking. A little bit nerdy too!