Whether you’re implementing agile development tips, refining your code deployment strategies, or optimizing your use of developer tools, this developer guide will equip you with the efficient coding techniques and secure coding practices necessary for success. As you progress, you’ll also discover version control best practices, software maintenance strategies, and how to foster a culture of continuous improvement within your development team.
Mastering the Developer-Lifecycle Relation: A Comprehensive Overview
Navigating the journey from an idea to a fully functional software application requires mastering the software development process. This comprehensive software development guide explores each phase of the software engineering lifecycle, offering developer best practices and coding standards that ensure efficiency and quality.
The journey from a mere idea to a fully functional application involves a complex process known as the software development lifecycle (SDLC). This encompasses several distinct phases:
Planning for Success: Requirement-Gathering and Goal-Setting
This is the foundational stage where the project’s blueprint is laid out.
This initial phase involves defining the developer’s goals, understanding the target audience, and pinpointing key features that will make the software product stand out.
- Conduct thorough market research: Analyze industry trends, competitor offerings, and target audience demographics using developer tools like Google Trends, SimilarWeb, and social media analytics.
- Gather user feedback: Utilize surveys, interviews, and usability testing to understand user needs and preferences. Create user feedback loops early in the software development process to refine requirements based on real-world insights.
- Create user personas: Develop fictional representations of target users to guide design and development decisions.
- Define project scope and goals: Clearly outline project objectives, features, and constraints.
For more on optimizing SDLC processes and setting up effective SLAs, check out our article on SDLC Success Strategies.
Designing with Precision: From Wireframes to Prototypes
Transforming abstract ideas into tangible designs is the focus of this phase.
Based on the gathered requirements, the design phase focuses on creating wireframes, mockups, and prototypes to visualize the software product’s user interface and user experience (UI/UX). This stage involves close collaboration between developers, designers, and stakeholders.
- Create wireframes: Develop skeletal outlines of the user interface, focusing on layout and content hierarchy.
- Design mockups: Visualize the application’s appearance using design tools like Figma, Adobe XD, or Sketch.
- Build interactive prototypes: Create clickable prototypes to simulate user interactions and gather feedback.
- User testing: Conduct usability tests to identify potential issues and make necessary refinements.
Coding Excellence: Writing Clean, Maintainable Code
This is where your coding skills take center stage.
This is where developers shine—writing the source code that transforms designs into functional applications, all while adhering to coding standards and developer best practices.
Developers write the code that brings the design to life, following coding standards and best practices. Version control systems like Git are essential for managing code changes efficiently.
- Choose the right technology stack: Choosing the right programming languages is crucial for writing maintainable and scalable software applications. Select frameworks and libraries that align with project requirements.
- Write clean, maintainable code: Adhere to coding standards and best practices to improve code readability and collaboration.
- Utilize version control: Utilize a version control system like Git to track code changes, collaborate effectively, and manage different source code versions, following version control best practices.
- Test-driven development (TDD): Write tests before writing code to ensure code quality and functionality. Implementing continuous delivery helps streamline the deployment process and reduces the risk of errors during production releases.
Make sure your development team collaborates closely to establish clear goals and efficient coding techniques for a successful project outcome.
Testing with Rigor: Ensuring Software Reliability
Thorough testing is crucial to ensure software quality and reliability.
Rigorous testing is crucial to identify and fix bugs before the software product is released. This phase involves various testing types, including unit testing, integration testing, and user acceptance testing (UAT).
- Unit testing: Verify the correct behavior of individual code components.
- Integration testing: Test how different parts of the application interact.
- End-to-end testing: Validate the entire application workflow from start to finish.
- Performance testing: Evaluate application speed, responsiveness, and scalability.
- Security testing: Identify vulnerabilities and protect against threats. Apply secure coding practices during development to prevent security vulnerabilities from compromising your application.
Deployment Done Right: Strategies for Seamless Launches
Deploying an application involves moving it from a development environment to a production environment.
Once the software product is thoroughly tested and approved, it’s time for code deployment strategies to take center stage. This involves moving the application from the development environment to a production environment, accessible to end-users.
- Choose a deployment strategy: Select a method like continuous deployment, continuous delivery, or release management.
- Configure deployment environments: Set up testing, staging, and production environments. Implement robust access controls during the deployment process to secure the production environment.
- Automate deployment: Use tools like Jenkins, Travis CI, or CircleCI to streamline the process.
- Monitor deployment health: Track metrics like uptime, response time, and error rates.
Security and compliance are also crucial at this stage. Learn more about this in our piece on The Compliance-Focused SDLC Tightrope.
Ongoing Maintenance: Keeping Your Code Base Strong
Software is a living product that requires ongoing care.
After deployment, the application requires ongoing maintenance, bug fixes, and updates to address new features or security vulnerabilities.
- Bug tracking and fixing: Implement a system to manage and prioritize bug reports as part of your software maintenance strategies.
- Feature updates: Plan and implement new features based on user feedback and market trends to continuously improve the software product.
- Security patches: Stay updated on vulnerabilities and apply necessary patches promptly.
- Performance optimization: Continuously monitor and improve application performance.
5 Essential Development Tools and Technologies Every Developer Should Master
To navigate the development process efficiently, developers rely on a vast array of tools and technologies:
Code Editors and IDEs
These tools provide a platform for writing and editing code, offering features like syntax highlighting, code completion, and debugging. Popular options include Visual Studio Code, Sublime Text, and IntelliJ IDEA.
Feature | Visual Studio Code | Sublime Text | IntelliJ IDEA |
Open-source |
Yes | No | No |
Platform Support |
Windows, macOS, Linux | Windows, macOS, Linux | Windows, macOS, Linux |
Language Support |
Extensive | Good | Excellent (especially for Java) |
Debugging |
Built-in | Extensions | Built-in |
Extensibility |
High | High | High |
Version Control Systems
Git is the industry standard for managing code changes, allowing developers to collaborate efficiently and track code history.
Feature |
Git | SVN | Mercurial |
Distributed vs. Centralized |
Distributed | Centralized | Distributed |
Branching Model |
Flexible | Less flexible | Flexible |
Popularity |
High | Medium | Low |
Learning Curve |
Moderate | Low | Medium |
Version control isn’t just about code management; it’s about enhancing collaboration. Discover how to empower your DevOps and SRE teams in our guide on Hiring and Empowering DevOps & SRE.
Build Tools
Tools like Grunt, Gulp, or Webpack automate repetitive tasks, such as compiling code, minifying files, and running tests.
Feature |
Grunt | Gulp | Webpack |
Focus |
Task automation | Task automation, streaming | Module bundling |
Language |
JavaScript | JavaScript | JavaScript |
Complexity |
Beginner-friendly | Intermediate | Advanced |
Ecosystem |
Large | Large | Very large |
Debugging Tools
These help developers identify and fix errors in their code, improving application stability.
Feature | Chrome DevTools | Visual Studio Debugger | gdb |
Browser-based |
Yes | No | No |
Platform Support |
Chrome, Edge | Windows, macOS | Linux, macOS, Unix-like systems |
Language Support |
JavaScript | C#, C++, Visual Basic | C, C++ |
Features |
Breakpoints, call stacks, console, network | Breakpoints, watch windows, call stacks | Breakpoints, watchpoints, command-line interface |
Deployment Platforms
Platforms like Heroku, AWS, Azure, and Google Cloud Platform offer infrastructure for deploying and hosting applications.
Feature |
Chrome DevTools | Visual Studio Debugger | gdb |
Browser-based |
Yes | No | No |
Platform Support |
Chrome, Edge | Windows, macOS | Linux, macOS, Unix-like systems |
Language Support |
JavaScript | C#, C++, Visual Basic | C, C++ |
Features |
Breakpoints, call stacks, console, network | Breakpoints, watch windows, call stacks | Breakpoints, watchpoints, command-line interface |
5 Best Practices for Efficient Development
Implement these core principles to streamline your development process and deliver high-quality software.
Adopting Agile to Boost Developer Efficiency
This iterative approach emphasizes flexibility, collaboration, and rapid development to boost customer satisfaction.
- Do: Break down projects into smaller, manageable iterations (sprints). Foster open communication and collaboration within the team. Prioritize customer feedback.
- Don’t: Rely solely on documentation. Ignore changes in requirements. Overemphasize individual contributions over team success.
Streamlining Your Workflow With Continuous Integration & Delivery
CI/CD is a set of practices that automate the building, testing, and deployment of code to improve efficiency and reduce errors.
- Do: Automate build, test, and deployment processes. Integrate with version control. Monitor pipeline performance.
- Don’t: Deploy code to production without thorough testing. Ignore pipeline failures. Neglect security checks in the pipeline.
Enhancing Developer Collaboration Through The Power of Code Reviews
Code reviews are essential for sharing knowledge. Peer reviews help improve code quality and identify potential issues.
- Do: Provide constructive feedback. Encourage code ownership. Use a code review tool.
- Don’t: Rush through code reviews. Be overly critical without offering solutions. Ignore code style guidelines.
Building a Solid Foundation for Your Code With Test-Driven Development
Test-driven development is a cornerstone of quality software. TDD is a development approach that involves writing tests before writing code to ensure code quality and functionality.
- Do: Write clear and concise tests. Refactor code based on test failures. Use a testing framework.
- Don’t: Write overly complex tests. Ignore test coverage. Neglect to update tests when code changes.
Explore how Agile methodologies can enhance your development process in our article on Agile Nearshore Development.
Mastering Version Control: Best Practices for Developers
Version control is essential for managing code changes. Use Git effectively to manage code changes, collaborate with team members, and track project history.
- Do: Commit code frequently with clear commit messages. Use branching effectively. Resolve merge conflicts promptly.
- Don’t: Commit large, untested code changes. Ignore code reviews. Force push changes.
Developer Guide on Overcoming Common Development Challenges
Developers often encounter obstacles throughout the development process. Some common challenges include:
Debugging and Troubleshooting
Identifying and fixing errors can be time-consuming.
- Leverage debugging tools: Utilize built-in debugger features, browser developer tools, or standalone debuggers to step through code execution.
- Implement logging: Add detailed logs to track code execution and identify issues.
- Practice rubber duck debugging: Explain the problem aloud to someone (or even a rubber duck) to gain a fresh perspective.
Meeting Deadlines
Balancing project requirements with time constraints can be stressful.
- Prioritize tasks: Focus on high-impact features first.
- Break down tasks: Divide large tasks into smaller, manageable subtasks. Effective time management is a critical skill for developers looking to meet deadlines without sacrificing quality.
- Effective time management: Utilize time tracking developer tools and time management techniques to enhance productivity within the development team. Adopting agile development tips helps your development team remain flexible and responsive to changes.
Code Optimization
Improving code performance and efficiency can be complex.
- Identify performance bottlenecks: Use profiling tools to pinpoint areas for improvement.
- Optimize algorithms and data structures: Choose efficient data structures and algorithms.
- Code refactoring: Improve code readability and maintainability while enhancing performance.
Security
Protecting software applications from security vulnerabilities is crucial.
- Follow security best practices: Adhere to coding standards and security guidelines. Regularly update your software engineering practices to align with the latest secure coding practices.
- Conduct regular security audits: Identify and address vulnerabilities.
- Use security frameworks and libraries: Leverage existing tools to protect your application. Developers must stay proactive with security measures to protect their code from vulnerabilities.
Dive deeper into security with our guide on SDLC Compliance Tools.
Collaboration
Effective communication and teamwork are essential for successful projects.
- Effective communication: Utilize collaboration tools like Slack, Teams, or Jira.
- Regular team meetings: Hold stand-ups or sprint planning meetings.
- Conflict resolution: Address disagreements and conflicts promptly and professionally. Incorporate continuous improvement in your software development process to adapt to evolving challenges and opportunities.
The Developer’s Path: Continuous Learning and Growth
The journey from zero to deployment is a complex but rewarding process. By understanding the development lifecycle, utilizing the right tools, and adopting best practices, developers can create high-quality applications efficiently.
Continuous learning and adaptation are key to staying ahead in the ever-evolving tech landscape. Reach out to Ubiminds and learn where we can take you to next.
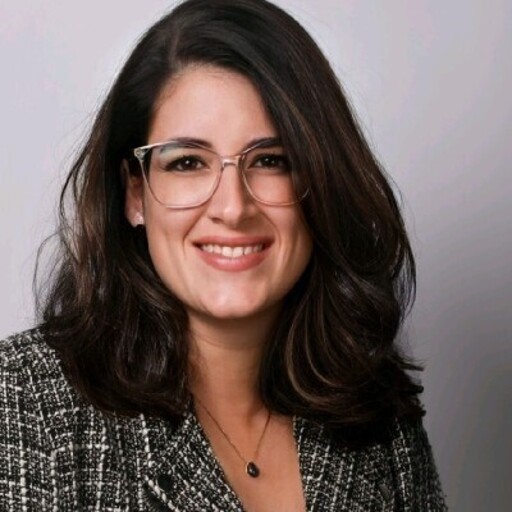
International Marketing Leader, specialized in tech. Proud to have built marketing and business generation structures for some of the fastest-growing SaaS companies on both sides of the Atlantic (UK, DACH, Iberia, LatAm, and NorthAm). Big fan of motherhood, world music, marketing, and backpacking. A little bit nerdy too!