Code mistakes can be costly. Learning how to fix code efficiently is crucial for any developer. This guide will help you avoid common coding errors, enhance your debugging skills, and implement coding best practices for a smoother workflow.
Coding Catastrophes: Common Mistakes and How to Fix Them
Avoiding common programming mistakes and writing clean, efficient code can (literally!) make or break your software. Remember: regularly debugging and refactoring code helps avoid common programming mistakes.
Mistake 1: Hardcoding Values
Hardcoding values directly into your code is a frequent code mistake that can make it rigid and difficult to maintain. It is one of the most frequent code mistakes that can lead to coding errors in large-scale applications.
Instead, use variables or constants to store values, making your code more flexible and easier to update.
Example:
JavaScript
// Hardcoded value
// Using a variable |
Mistake 2: Using Overly Complex Logic
Using overly complex logic is one of the common programming mistakes that can make your code difficult to understand and maintain.
To fix errors in your logic, consider simplifying your approach by breaking down tasks into smaller, more manageable functions. Use functions and modules to organize your code and improve readability.
Example:
Python
# Complex logic # Simplified logic |
Mistake 3: Neglecting Code Formatting and Style
Consistent formatting isn’t just about aesthetics; it’s a critical part of coding best practices that helps avoid subtle coding errors and improves collaboration.
Following coding best practices like consistent formatting and style make code easier to read and understand. Adhere to coding conventions and use tools like linters to automatically enforce style rules.
Example:
JavaScript
// Poor formatting // Good formatting |
Mistake 4: Ignoring Error Handling
Implementing error handling in code is crucial for preventing and mitigating coding errors before they escalate into bigger problems. Thus, it creates robust and reliable software.
Use try-catch blocks to catch exceptions and provide informative error messages.
Example:
Python
try: |
Mistake 5: Not Using Version Control
Version control is also vital when you need to fix errors or revert to a previous state after discovering a significant code mistake.
Version control systems like Git help you track changes to your code, collaborate effectively, and revert to previous versions if needed. Not using version control is a critical coding error that can compromise the health of your codebase.
Example:
Bash
git init |
Coding Best Practices
To write clean, maintainable code, it’s important to follow coding best practices that not only improve readability but also help you quickly fix errors when they arise. Incorporating thorough error handling in code ensures that your application can gracefully manage unexpected conditions and continue to function smoothly. We suggest you:
- Write clear and concise code comments.
- Use meaningful variable names.
- Test your code thoroughly.
- Review and refactor code regularly.
- Stay updated with coding best practices and emerging technologies.
Quick FAQ for Code Mistakes
- What are common code mistakes?
Common code mistakes include hardcoding values, using overly complex logic, neglecting error handling, and ignoring version control. - How can I fix code mistakes effectively?
To effectively fix code mistakes, break down complex logic, use version control, handle errors properly, and follow consistent coding practices. - Why is error handling important in coding?
Error handling ensures that your software can gracefully manage unexpected situations, leading to more robust and reliable applications. - What tools can help reduce coding mistakes?
Tools like linters, version control systems like Git, and IDEs with syntax highlighting can help reduce coding errors and improve code quality.
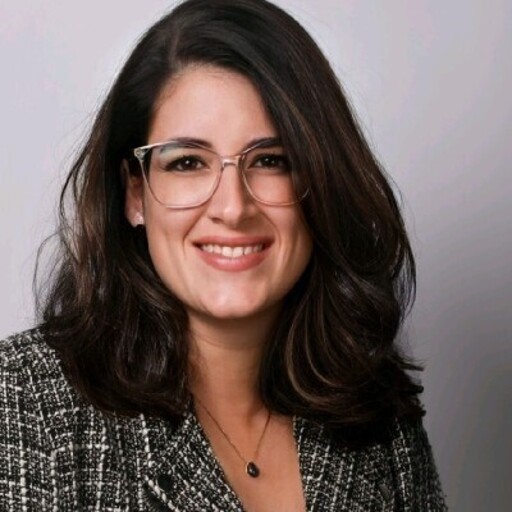
International Marketing Leader, specialized in tech. Proud to have built marketing and business generation structures for some of the fastest-growing SaaS companies on both sides of the Atlantic (UK, DACH, Iberia, LatAm, and NorthAm). Big fan of motherhood, world music, marketing, and backpacking. A little bit nerdy too!