Even the most skilled developers can encounter a code error. Understanding and fixing these coding mistakes is essential for code quality and maintaining software integrity. This guide will help you identify common syntax errors, logic errors, and performance issues, providing solutions that align with software development best practices.
Mastering Code Quality
A successful software project requires careful planning, clear communication, and adherence to coding standards. That’s why adopting a uniform coding style across the team helps to avoid confusion and makes onboarding new developers easier.
- Developers need to account for different operating systems when building cross-platform applications. Effective software engineering combines technical skills with problem-solving to create robust and scalable applications.
- Every line of code should serve a purpose; unnecessary lines can lead to bloated and inefficient software.
- Always test every piece of code you write to ensure it works as expected before merging it into the main branch.
Adhering to well-defined coding guidelines ensures consistency and maintainability across the team. Consistency in formatting improves readability, making it easier for others to understand and maintain the code.
Avoiding Common Code Errors
Understanding common code errors helps developers improve code quality and adhere to software development best practices.
Syntax Errors
Syntax errors occur when the code violates the grammatical rules of the programming language. They are typically caught by the compiler or interpreter during compilation or runtime. Look for error messages that indicate incorrect syntax, such as missing or misplaced punctuation, incorrect variable names, or unbalanced parentheses.
Common Syntax Errors
- Missing or misplaced punctuation: Ensure proper use of parentheses, brackets, and semicolons.
- Incorrect variable names or types: Verify that variable names are valid and that data types are used correctly.
- Unbalanced parentheses or brackets: Check for matching pairs of parentheses and brackets.
How to Prevent and Fix Syntax Errors
- Use a code editor with syntax highlighting: This can help you visually identify syntax errors.
- Follow coding conventions: Adhere to the style guide for your programming language to maintain consistency and readability.
- Test your code thoroughly: Run your code through a linter or static code analyzer to catch syntax errors early.
Logic Errors
Logic errors occur when the code executes correctly but produces incorrect results due to flawed logic or algorithms. Test your code with various inputs and compare the output to the expected results. Use debugging tools to step through your code and identify where the logic goes wrong.
Common Logic Errors
- Incorrect conditional statements: Ensure that conditions are evaluated correctly and that the appropriate code branches are executed.
- Infinite loops: Avoid creating loops that never terminate.
- Off-by-one errors: Pay attention to array indices and loop boundaries to prevent errors.
How to Prevent and Fix Logic Errors
- Write clear and concise code: Use meaningful variable names and comments to improve code readability.
- Test thoroughly: Create comprehensive test cases to cover different scenarios and edge cases.
- Use debugging tools: Utilize debuggers to step through your code and inspect variable values.
Runtime Errors
Runtime errors occur when the code executes without syntax errors but encounters an error during runtime. Look for error messages that indicate runtime exceptions or crashes. Use a debugger to examine the call stack and identify the source of the error.
Common Runtime Errors
- Null pointer exceptions: Handle null references gracefully to prevent unexpected crashes.
- Array out of bounds errors: Ensure that array indices are within the valid range.
- Division by zero: Avoid dividing by zero, as it can lead to runtime errors.
How to Prevent and Fix Runtime Errors
- Handle exceptions: Use try-catch blocks to handle potential exceptions and prevent your program from crashing.
- Validate input: Check user input for validity and prevent unexpected behavior.
- Use defensive programming techniques: Write code that is resilient to errors and unexpected conditions.
Performance Issues
Performance issues occur when code is inefficient or consumes excessive resources. Use profiling tools to measure the performance of your code and identify bottlenecks.
Common Performance Issues
- Inefficient algorithms: Choose algorithms that have a lower time complexity for large datasets.
- Unnecessary computations: Avoid redundant calculations and optimize your code for efficiency.
- Excessive memory usage: Minimize memory allocation and deallocate resources when no longer needed.
How to Prevent and Code Performance Issues
- Optimize algorithms: Choose efficient algorithms and data structures.
- Reduce unnecessary computations: Avoid redundant calculations and optimize your code for efficiency.
- Minimize memory usage: Manage memory allocation and deallocation effectively.
Security Vulnerabilities
Security vulnerabilities can expose your application to attacks and compromise user data. Conduct security audits and vulnerability assessments to identify potential weaknesses.
Common Security Vulnerabilities
- Injection attacks: Protect against SQL injection, cross-site scripting (XSS), and other injection vulnerabilities.
- Cross-site request forgery (CSRF): Implement CSRF prevention measures to protect against unauthorized actions.
- Sensitive data exposure: Handle sensitive data securely and avoid exposing it to unauthorized parties.
How to Prevent and Fix Security Vulnerabilities
- Follow security best practices: Adhere to security guidelines and standards for your programming language and framework.
- Use secure coding practices: Avoid common security vulnerabilities like SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF).
- Keep your dependencies updated: Regularly update libraries and frameworks to address known security vulnerabilities.
Common Code Errors in Agile Development
Agile development emphasizes quick iterations and collaboration among team members. It’s crucial to implement coding standards and automated testing to catch coding mistakes early and improve code quality.
The Importance of Code Reviews
Regular code reviews by peers are essential in catching coding errors and improving clean code practices. Feedback from team members enhances overall team performance and minimizes technical debt.
Language-Specific Mistakes
Different programming languages have their own unique characteristics and behaviors that can lead to specific coding mistakes. Understanding these nuances is crucial for developers aiming to enhance code quality, avoid performance issues, and prevent security vulnerabilities. Below are common pitfalls along with explanations and best practices for avoiding these mistakes.
Python
Python’s reliance on indentation for block definitions can lead to errors if not managed carefully. Additionally, its handling of mutable objects can introduce unintended side effects.
- Indentation Errors: In Python, consistent indentation is not just a matter of style; it defines the structure of the code. Improper indentation can lead to syntax errors, making the code difficult to read and maintain. Always use spaces (or tabs) consistently and adhere to the PEP 8 style guide to ensure clarity and reduce the risk of misinterpretation by both the interpreter and other developers.
- Mutable Default Arguments: When using mutable default arguments (like lists or dictionaries) in function definitions, be aware that these defaults are shared across all calls to the function. This can lead to unexpected behavior and hard-to-trace bugs. To avoid this, use immutable types (like None) as defaults and create a new mutable object within the function.
JavaScript
JavaScript’s flexible nature can lead to subtle bugs, especially with its asynchronous programming model and variable hoisting behavior.
- Hoisting: In JavaScript, variable and function declarations are hoisted to the top of their containing scope. This can lead to code errors if developers are unaware of the hoisting behavior, which may cause variables to be undefined when accessed. Always declare variables at the top of their scope and use let or const instead of var to avoid confusion regarding scoping rules.
- Asynchronous Programming: Handling asynchronous operations can introduce complexities like race conditions and callback hell. It’s essential to use modern asynchronous patterns, such as async/await, to simplify code flow and improve code quality. This helps in managing asynchronous operations more effectively and maintaining readability.
Java
Java’s strong type system and memory management features present their own set of challenges, particularly around object references and resource allocation.
- Null Pointer Exceptions: These occur when a program attempts to access an object that has not been initialized. Implementing null checks or using the Optional class can mitigate these common coding errors. Always validate object references before use to enhance overall code quality.
- OutOfMemoryError: This error happens when the Java Virtual Machine (JVM) runs out of memory. To prevent it, manage memory usage by monitoring object creation and ensuring that unnecessary references are cleared, thus allowing garbage collection to reclaim memory effectively.
C++
C++ provides powerful capabilities but also demands careful memory management and awareness of language intricacies to avoid bugs.
- Memory Leaks: In C++, failing to deallocate memory can lead to performance issues as memory consumption increases over time. Always pair every new with a corresponding delete to prevent leaks. Additionally, consider using smart pointers (e.g., std::unique_ptr or std::shared_ptr) to automate memory management and reduce the risk of leaks.
- Undefined Behavior: C++ allows for operations that can lead to unpredictable results, such as accessing out-of-bounds array elements or using uninitialized variables. To avoid this, implement robust error handling and employ static analysis tools to detect potential undefined behavior during development.
C#
C# combines object-oriented programming with a robust type system, but it can introduce errors if best practices are not followed.
- Null Reference Exceptions: These exceptions occur when accessing members on a null object reference. In C#, this is a common pitfall that can disrupt program execution. To mitigate this, use nullable reference types (introduced in C# 8.0) to indicate when null values are permissible. Additionally, implementing null checks and employing the null-coalescing operator can help prevent these exceptions.
- Async/Await Misuse: Misunderstanding the async/await model can lead to deadlocks or inefficient task management. It’s essential to ensure that asynchronous methods are configured correctly, avoiding blocking calls within async methods. Properly leveraging Task.Run and asynchronous programming patterns can enhance performance and maintainability.
Ruby
Ruby’s dynamic nature allows for rapid development but can also result in certain pitfalls.
- Type Errors: Ruby is dynamically typed, meaning that type errors are often only caught at runtime. This flexibility can lead to unexpected behavior if types are not carefully managed. Implement thorough unit tests to catch potential type-related issues early in the development cycle. Utilizing type-checking tools like Sorbet can help enforce type contracts and enhance code reliability.
- Global State Modification: Modifying global variables can lead to hard-to-trace bugs and unpredictable behavior in Ruby applications. To avoid this pitfall, limit the use of global state by employing encapsulation and modular design patterns. Use instance variables or class methods to encapsulate state within objects, enhancing maintainability and clarity.
Preventing Common Coding Errors: 4 Best Practices for Developers
Preventing common coding errors is crucial for maintaining high-quality code and enhancing overall project efficiency. Adopting effective practices can significantly reduce the likelihood of bugs, improve team collaboration, and lead to a more robust software product. Here are some key strategies to consider:
#1 Code Reviews
Implementing a systematic code review process is one of the most effective ways to identify errors and enhance code quality. By having peers review your code, you can benefit from different perspectives and expertise, leading to more robust solutions.
That’s why every change should go through pull requests to ensure quality and encourage peer review.
- Peer Insights: Engaging in code reviews promotes knowledge sharing among team members, fostering a culture of collaboration. Reviewers can catch potential bugs, suggest more efficient algorithms, and help ensure adherence to coding standards.
- Constructive Feedback: Aim to create a supportive environment where feedback is constructive. This encourages developers to improve their skills and write better code in the future.
- Review Tools: Leverage code review tools like GitHub, Bitbucket, or GitLab that facilitate discussions and streamline the review process. These platforms allow for inline comments and version comparisons, making it easier to track changes and resolve issues collaboratively.
#2 Continuous Learning
The technology landscape is constantly evolving, and staying updated on best practices and emerging technologies is vital for avoiding outdated or inefficient coding techniques.
- Stay Informed: Regularly read industry blogs, participate in webinars, and follow thought leaders in software development. This helps you stay abreast of new languages, frameworks, and methodologies.
- Formal Education: Consider enrolling in online courses or workshops that focus on modern programming practices and new technologies. Certifications in specific tools or languages can also bolster your skill set and improve job prospects.
- Community Engagement: Join developer communities and forums to engage in discussions, ask questions, and share experiences. Platforms like Stack Overflow, GitHub, and local meetups can provide valuable insights and support.
#3 Utilize Code Analysis Tools
Incorporating static code analysis tools into your development workflow can help detect potential issues early in the coding process and enforce coding standards.
- Automated Checks: Tools like SonarQube, ESLint, or Checkstyle can automatically analyze your codebase for common errors, security vulnerabilities, and code smells. This automation saves time and reduces the cognitive load on developers.
- Customization: Customize these tools to align with your project’s specific coding standards and best practices. Establishing rules and guidelines that fit your team’s workflow will enhance their effectiveness.
- Continuous Integration (CI): Integrate code analysis tools into your CI pipeline to ensure that code is automatically checked for issues during the build process. This integration can catch errors before they reach production, reducing the likelihood of bugs in the final product.
Automating testing and deployment through CI/CD pipelines accelerates development and reduces the chances of human error.
#4 Learn from Mistakes
Every developer makes mistakes; what matters is how you respond to them. Analyzing past errors is essential for growth and improving future coding practices.
- Post-Mortem Analysis: Conduct post-mortem reviews for significant bugs or issues. Discuss what went wrong, identify contributing factors, and document lessons learned to prevent similar issues in the future.
- Error Tracking: Utilize error tracking tools like Sentry or Rollbar to monitor and log exceptions in your applications. This allows you to analyze patterns in errors and address underlying problems effectively.
- Foster a Growth Mindset: Cultivate an environment where mistakes are viewed as learning opportunities rather than failures. Encourage developers to share their experiences and insights, leading to a culture of continuous improvement.
Emphasizing collaboration, ongoing education, automated tools, and reflection on past experiences will create a more resilient and skilled development team. By understanding and addressing these common coding mistakes, you can write more robust, efficient, and secure code. Regular code reviews, testing, and continuous learning can also help you improve your coding practices and avoid future errors.
FAQ
- What is a coding error? A coding error is a mistake in the code that can lead to unexpected behavior, performance issues, or security vulnerabilities.
- How can I identify coding errors? Use debugging tools, code reviews, and testing to identify coding errors.
- How can I prevent coding errors? Follow coding best practices, write clean and readable code, and test your code thoroughly.
- What are the most common coding mistakes? Common coding mistakes include syntax errors, logic errors, runtime errors, performance issues, and security vulnerabilities.
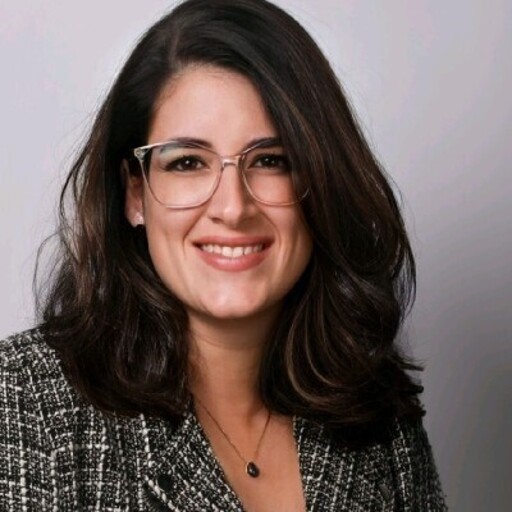
International Marketing Leader, specialized in tech. Proud to have built marketing and business generation structures for some of the fastest-growing SaaS companies on both sides of the Atlantic (UK, DACH, Iberia, LatAm, and NorthAm). Big fan of motherhood, world music, marketing, and backpacking. A little bit nerdy too!